Write a NeXus HDF5 file¶
In this example, the 1-D scan data will be written into the simplest
possible NeXus HDF5 data file, containing only the required NeXus components.
NeXus requires at least one NXentry group at the root level of
an HDF5 file. The NXentry
group contains all the data and associated
information that comprise a single measurement.
NXdata
is used to describe the
plottable data in the NXentry
group. The simplest place to store
data in a NeXus file is directly in the NXdata
group,
as shown in the next figure.
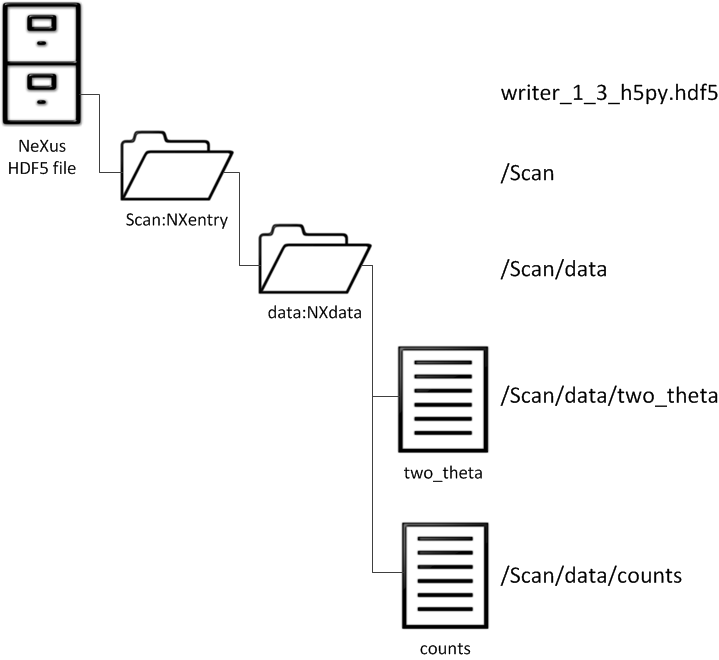
Simple Example¶
In the above figure,
the data file (simple_example_write1_h5py.hdf5
) contains
a hierarchy of items, starting with an NXentry
named entry
.
(The full HDF5 path reference, /entry
in this case, is shown to the right of each
component in the data structure.) The next h5py
code
example will show how to build an HDF5 data file with this structure.
Starting with the numerical data described above,
the only information
written to the file is the absolute minimum information NeXus requires.
In this example, you can see how the HDF5 file is created, how
Groups and datasets (Fields)
are created, and how Attributes are assigned.
Note particularly the NX_class
attribute on each HDF5 group that
describes which of the NeXus Base Class Definitions is being used.
When the next Python program (simple_example_write1_h5py.py
) is run from the
command line (and there are no problems), the simple_example_write1_h5py.hdf5
file is generated.
1#!/usr/bin/env python
2"""
3Writes the simplest NeXus HDF5 file using h5py
4
5Uses method accepted at 2014NIAC
6according to the example from Figure 1.3
7in the Introduction chapter
8"""
9
10from pathlib import Path
11
12import numpy
13
14from nexusformat.nexus import NXdata, NXentry, NXfield, nxopen
15
16filename = str(Path(__file__).absolute().parent.parent / "simple_example.dat")
17buffer = numpy.loadtxt(filename).T
18tthData = buffer[0]
19countsData = numpy.asarray(buffer[1], "int32")
20
21with nxopen("simple_example_write1.hdf5", "w") as f: # create the NeXus file
22 f["Scan"] = NXentry()
23 tth = NXfield(tthData, name="two_theta", units="degrees")
24 counts = NXfield(countsData, name="counts", units="counts")
25 f["Scan/data"] = NXdata(counts, tth)
1#!/usr/bin/env python
2"""
3Writes the simplest NeXus HDF5 file using h5py
4
5Uses method accepted at 2014NIAC
6according to the example from Figure 1.3
7in the Introduction chapter
8"""
9
10from pathlib import Path
11import h5py
12import numpy
13
14filename = str(Path(__file__).absolute().parent.parent / "simple_example.dat")
15buffer = numpy.loadtxt(filename).T
16tthData = buffer[0] # float[]
17countsData = numpy.asarray(buffer[1], "int32") # int[]
18
19with h5py.File("simple_example_write1.hdf5", "w") as f: # create the HDF5 NeXus file
20 # since this is a simple example, no attributes are used at this point
21
22 nxentry = f.create_group("Scan")
23 nxentry.attrs["NX_class"] = "NXentry"
24
25 nxdata = nxentry.create_group("data")
26 nxdata.attrs["NX_class"] = "NXdata"
27 nxdata.attrs["signal"] = "counts"
28 nxdata.attrs["axes"] = "two_theta"
29 nxdata.attrs["two_theta_indices"] = [
30 0,
31 ]
32
33 tth = nxdata.create_dataset("two_theta", data=tthData)
34 tth.attrs["units"] = "degrees"
35
36 counts = nxdata.create_dataset("counts", data=countsData)
37 counts.attrs["units"] = "counts"
One of the tools provided with the HDF5 support libraries is
the h5dump
command, a command-line tool to print out the
contents of an HDF5 data file. With no better tool in place (the
output is verbose), this is a good tool to investigate what has been
written to the HDF5 file. View this output from the command line
using h5dump simple_example_write1.hdf5
. Compare the data contents with
the numbers shown above. Note that the various HDF5 data types have all been
decided by the h5py
support package.
Note
The only difference between this file and one written using the NAPI is that the NAPI file will have some additional, optional attributes set at the root level of the file that tells the original file name, time it was written, and some version information about the software involved.
Since the output of h5dump
is verbose (see the Downloads section below),
the punx tree tool [1] was used to
print out the structure of HDF5 data files. This tool provides a simplified view
of the NeXus file. Here is the output:
1 Scan:NXentry
2 @NX_class = "NXentry"
3 data:NXdata
4 @NX_class = "NXdata"
5 @axes = "two_theta"
6 @signal = "counts"
7 @two_theta_indices = [0]
8 counts:NX_INT32[31] = [1037, 1318, 1704, '...', 1321]
9 @units = "counts"
10 two_theta:NX_FLOAT64[31] = [17.92608, 17.92591, 17.92575, '...', 17.92108]
11 @units = "degrees"
As the data files in these examples become more complex, you will appreciate the information density provided by punx tree.
downloads¶
The Python code and files related to this section may be downloaded from the following table.
file |
description |
---|---|
2-column ASCII data used in this section |
|
h5py code to write example simple_example_write1 |
|
nexusformat code to write example simple_example_write1 |
|
NeXus file written by this code |
|
h5dump analysis of the NeXus file |
|
punx tree analysis of the NeXus file |